Lets start by the Title Menu
Ok so first of all lets talk about what I've ready so I can bring y'all up to speed. I'm going to start with the title menu:
This is an image of the menu, lots of things need to be improved in the display but my focus was to make it functional as fast as possible. The selected option of this menu is the one and on selection a function is called. This menu is implemented as a finite state machine where the menu options are the states and the keyboard keys activate transitions; and these transition have functions attached.
When a key is pressed the menu option changes to another menu option (that can be the same as the first one). The transitions on this menu are press "down" to move to the option below, "up" to move to the option above and "z" to move to the same option and activate the option functionality.
Basically I used this complicated way to say "down" goes down, "up" goes up and when you select an option with "z" stuff happens. So why I did this to myself? The reason is because I can build any type of menu I want with this foundation.
For example lets take the Super Smash Bros Brawl Menu:
In this image we are in the "Group" option. The transitions for these option with what we mentioned would be "right" to go to the "Solo" option, "down" to go to the "Wifi" option and finally "2" go to the group menu (if we use a wii mote). We can do that for all options and boom, we have a menu. That is just a small taste of the true power and versatility of finite states machines.
But you may be asking: "Isn't it too tedious to set manually all transitions of the menu of your game given that for all options 'up' is go up, 'down' is go down and 'z' activates the option's function?", "What about dynamic menues huh??". The answer to all of these questions is Builder Pattern.
The Builder Pattern is a code design pattern that helps us create complex stuff step by step in code. In this case I made a builder class where the code looked something like this:
class TitleMenuBuilder{ function constructor(){ // Create an empty array of menu states this.menu_states = [] } // adds a new option to the menu function addStateWithFunction(state_function, option_name){ // Create a new menu state state = MenuState.new(option_name) // Create the activation transition state.addTransition(key = "z", next_state = state, transition_function = state_function ) // Creates the up and down transitions with the last state of the menu (null function is a function that // does nothing) state.addTransition(key="up", next_state = this.getLastState(), transition_function = null_function ) this.getLastState().addTransition(key="down", next_state = state, transition_function = null_function ) this.menu_states.append(state) // This last part is convention return this } // Returns the menu object function getMenu(){ return Menu.new(this.menu_states) } }
Using this baby creating the title menu was a piece of cake and was also used when creating the pause menu prototype:
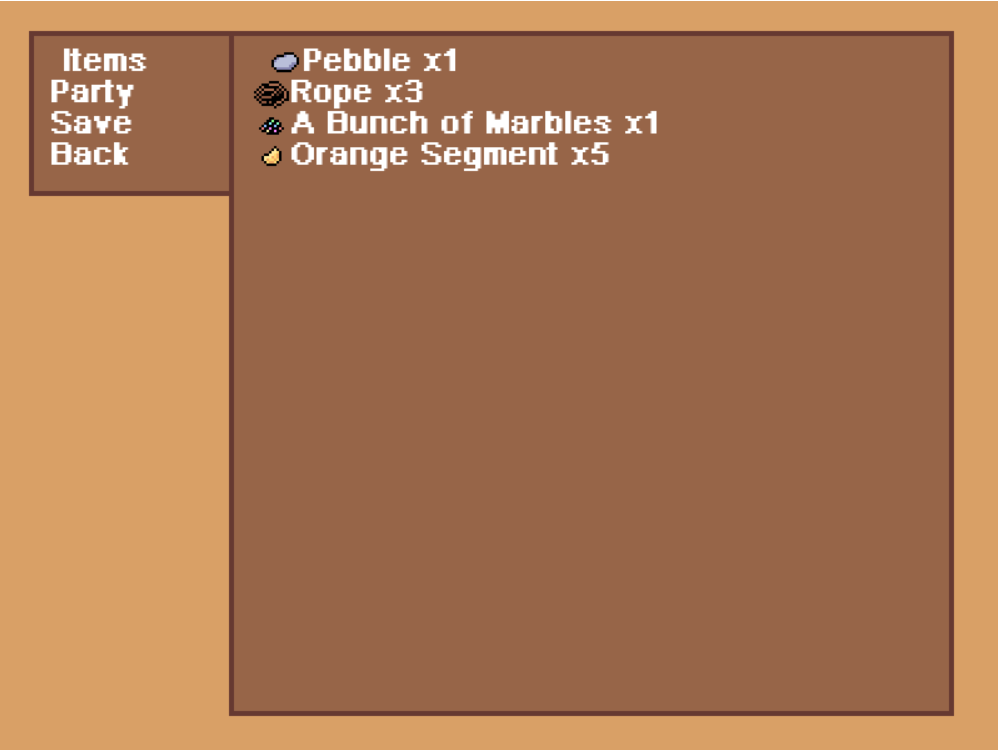
Thats all for this post :D
Get The Runemaster's Diary
The Runemaster's Diary
A small turn-based rpg about a kid and an orange trying to save a dog.
Status | In development |
Author | Tomyatemo |
Genre | Role Playing |
Tags | 2D, JRPG, Pixel Art, Short, Top-Down, Top Down Adventure, Turn-Based Combat |
More posts
- Devlog September 2023 (FINALE??)Sep 28, 2023
- Devlog August 2023Sep 01, 2023
- Devlog July 2023Jul 28, 2023
- Devlog June 2023Jul 10, 2023
- Devlog May 2023May 29, 2023
- Hotfixes Demo #2May 13, 2023
- Hotfixes Demo #1May 05, 2023
- First Devlog of 2023! (Devlog April)May 04, 2023
- Devlog JuneJul 05, 2022
- Devlog MayMay 31, 2022
Leave a comment
Log in with itch.io to leave a comment.